Django Meets Instagram API: A Tech Voyage 🚢🌐
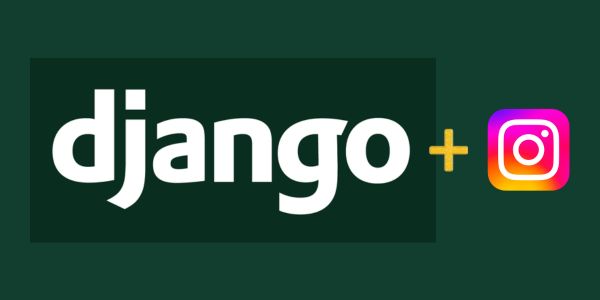
Ahoy, tech adventurers! 🙋♂️✨ Today, let’s set sail across the vast digital oceans and anchor our Django ship onto the shores of Instagram’s treasures. 🌍📸 Ready? Let’s cast away!
1. Preparing for the Voyage: The Setup 🧳🧭
Like all legendary quests, ours starts with proper preparation:
- First and foremost, carve out a niche on the Facebook Developer platform. It’s like getting a nifty map for our journey. 🗺️
- Secure the golden keys: the access tokens. Guard them fiercely; they’re as precious as a dragon’s hoard! 🐉🔐
2. Enlisting Our First Mate: The Requests Library 🦜
Even the most skilled captain needs a trustworthy first mate. For us, it’s the requests
library, our bridge to distant lands:
pip install requests
3. Building Our Mighty Vessel: The Implementation 🌊🛠️
With our compass set and first mate onboard, let’s construct our ship!
3.1 Model: The Ship’s Hull 🛶
Our vessel’s backbone! It will carry the treasures – Instagram posts – from far-off lands:
# models.py from django.db import models class InstagramPost(models.Model): post_id = models.CharField(max_length=100, unique=True) caption = models.TextField(blank=True, null=True) image_url = models.URLField(max_length=500) comments = models.TextField() # We're storing as a JSON string for now. Fancy, right?
Each attribute here is a compartment, storing different gems 💎 – post IDs, captions, images, and comments.
3.2 Serializer: The Ship’s Scribe 📜✍️
For every treasure we collect, our ship’s scribe documents its story:
# serializers.py from rest_framework import serializers from .models import InstagramPost class InstagramPostSerializer(serializers.ModelSerializer): class Meta: model = InstagramPost fields = '__all__'
This helps translate the tales of our finds into legendary sagas understood by all. 📖
3.3 View: The Crow’s Nest 🦉
From the crow’s nest, we spot and collect treasures:
# views.py from rest_framework.views import APIView from rest_framework.response import Response from rest_framework import status import requests import json from .models import InstagramPost from .serializers import InstagramPostSerializer class FetchInstagramPosts(APIView): def get(self, request): # Your access token from Instagram API access_token = 'YOUR_ACCESS_TOKEN' try: # Fetch latest 10 posts url = f"https://graph.instagram.com/me/media?fields=id,caption,media_type,media_url,thumbnail_url,permalink,timestamp&access_token={access_token}&limit=10" response = requests.get(url) response.raise_for_status() # This will raise an HTTPError if the HTTP request returned an unsuccessful status code data = response.json().get('data', []) for post in data: # Only consider image posts for simplicity if post['media_type'] == 'IMAGE': post_id = post['id'] # Fetch comments for this post comments_url = f"https://graph.instagram.com/{post_id}/comments?fields=text,username&access_token={access_token}" comments_response = requests.get(comments_url) comments_response.raise_for_status() comments_data = comments_response.json().get('data', []) # Create or update the post in our database InstagramPost.objects.update_or_create( post_id=post_id, defaults={ 'caption': post.get('caption', ""), 'image_url': post.get('media_url', ""), 'comments': json.dumps(comments_data) } ) # Return latest posts from our database posts = InstagramPost.objects.all() serializer = InstagramPostSerializer(posts, many=True) return Response(serializer.data, status=status.HTTP_200_OK) except requests.RequestException as e: # This is the base exception class for Requests. It will catch any network-related errors. return Response({'detail': f"Network error: {e}"}, status=status.HTTP_503_SERVICE_UNAVAILABLE) except json.JSONDecodeError: # Error decoding the JSON return Response({'detail': "Failed to decode the response from Instagram API"}, status=status.HTTP_500_INTERNAL_SERVER_ERROR) except Exception as e: # General error handler - catch all unexpected errors return Response({'detail': f"An unexpected error occurred: {e}"}, status=status.HTTP_500_INTERNAL_SERVER_ERROR)
From fetching posts to gathering comments, this is where the magic of exploration happens! 🔍✨
3.4 URL: The Ship’s Anchor ⚓
Our ship needs to dock, and the anchor ensures we’re always moored right:
# urls.py from django.urls import path from .views import FetchInstagramPosts urlpatterns = [ path('instagram/posts/', FetchInstagramPosts.as_view(), name='instagram_posts'), ]
4. Charting The Course: Additional Notes 🌌
- Our ship, though majestic, is a basic build. We haven’t yet equipped it for stormy seas (error handling), whirlpools (pagination), or pesky seagulls (rate limits) of the Instagram API.
- Guard your access tokens like a Kraken guards its lair! Never let them wander into the wild.
- The seven seas are vast and ever-changing; similarly, Instagram’s Graph API is evolving. Keep an eye on the official scrolls for updates.
And there we have it, brave adventurers! Our ship is ready, the compass set, and vast digital oceans await. 🌍🌊 May your code be your wind and your logic the guiding star. ⭐️
Until the next tide brings us together, sail on and code fearlessly! 🚢💖🎉