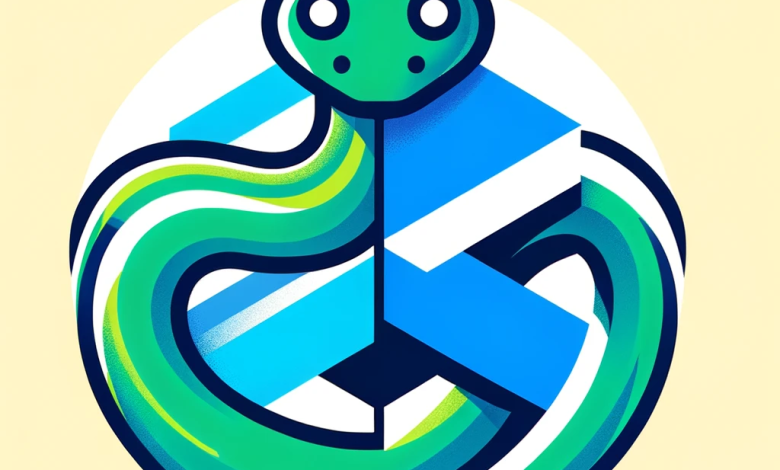
Building a dynamic and visually appealing FAQ page can greatly enhance user experience on your website. This guide will walk you through creating a chat-style FAQ layout using Django for the back-end and Bootstrap 5 for the front-end, including images next to questions and answers.
Setting Up the Back-end with Django
- Initialize Django Project: Install Django and create a new project and app.
- Define FAQ Model: Create a Django model to handle FAQ data, with fields for both questions and answers.
- Admin Interface: Use Django’s admin interface for easy management of FAQ entries.
- FAQ View: Develop a view in Django to retrieve FAQ data and pass it to the template.
Front-end Design with Bootstrap 5
- FAQ Template Design: Use Bootstrap 5 to design a responsive and organized FAQ layout. Incorporate Bootstrap’s grid system for alignment.
- Integrate Images with Text: Enhance visual appeal by adding images next to questions and answers using Bootstrap column classes.
- Custom CSS: Apply custom styling for well-aligned and appropriately sized images and text.
Code Implementation
Django FAQ Model (faq/models.py
)
from django.db import models class FAQ(models.Model): question = models.TextField() answer = models.TextField() def __str__(self): return self.question
Django FAQ View (faq/views.py
)
from django.shortcuts import render from .models import FAQ def faq_view(request): faqs = FAQ.objects.all() return render(request, 'faq.html', {'faqs': faqs})
Bootstrap 5 FAQ Template (faq/templates/faq.html
)
<!DOCTYPE html> <html> <head> <title>FAQ</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/5.0.0-alpha1/css/bootstrap.min.css"> <style> .faq-row { align-items: center; } .faq-image { max-width: 50px; } </style> </head> <body> <div class="container"> <h1>FAQ</h1> {% for faq in faqs %} <div class="row faq-row mb-3"> <div class="col-2 text-right"> <img src="path/to/question-image.jpg" class="faq-image"> </div> <div class="col-10"> <div class="alert alert-primary" role="alert">Q: {{ faq.question }}</div> </div> </div> <div class="row faq-row mb-3"> <div class="col-10"> <div class="alert alert-secondary" role="alert">A: {{ faq.answer }}</div> </div> <div class="col-2"> <img src="path/to/answer-image.jpg" class="faq-image"> </div> </div> {% endfor %} </div> </body> </html>
Conclusion
With Django‘s robust back-end functionality and Bootstrap 5‘s aesthetic front-end components, you can create an interactive and engaging FAQ section for your website. This approach combines functionality with visual appeal, making it an ideal choice for modern web applications.
I don’t even understand how I ended up right here, however I
thought this submit was once good. I don’t know who you might be but definitely you’re going to
a famous blogger if you aren’t already. Cheers!
I’m impressed, I have to admit. Rarely do I encounter a blog that’s
both equally educative and engaging, and let me tell you, you’ve hit the nail on the head.
The problem is an issue that not enough folks are speaking intelligently about.
Now i’m very happy I found this in my hunt for something regarding this.
Hey! This is my first visit to your blog! We are a team of
volunteers and starting a new initiative in a community in the same niche.
Your blog provided us beneficial information to work on. You have done a marvellous job!
It is perfect time to make some plans for the future and it is time to be happy.
I have read this post and if I could I wish to suggest you some interesting things or tips.
Perhaps you can write next articles referring to this article.
I wish to read even more things about it!