Python
Django & JS: Suggest Usernames Efficiently
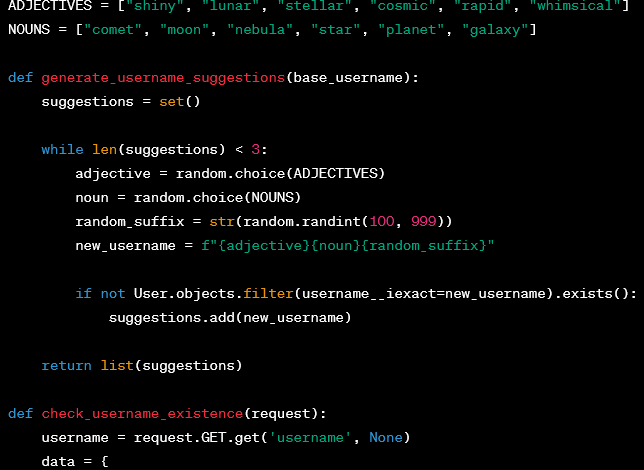
Create a list of adjectives and nouns.
If the username is taken, combine an adjective with a noun and possibly a random number to generate a suggestion.
Django: Modify the view for checking username existence and suggesting better alternatives:
# views.py from django.http import JsonResponse from django.contrib.auth.models import User import random ADJECTIVES = ["shiny", "lunar", "stellar", "cosmic", "rapid", "whimsical"] NOUNS = ["comet", "moon", "nebula", "star", "planet", "galaxy"] def generate_username_suggestions(base_username): suggestions = set() while len(suggestions) < 3: adjective = random.choice(ADJECTIVES) noun = random.choice(NOUNS) random_suffix = str(random.randint(100, 999)) new_username = f"{adjective}{noun}{random_suffix}" if not User.objects.filter(username__iexact=new_username).exists(): suggestions.add(new_username) return list(suggestions) def check_username_existence(request): username = request.GET.get('username', None) data = { 'is_taken': User.objects.filter(username__iexact=username).exists() } if data['is_taken']: data['suggestions'] = generate_username_suggestions(username) return JsonResponse(data)
Use JavaScript to send an AJAX request
Using vanilla JavaScript:
function checkUsernameExistence(username) { fetch("/check_username_existence/?username=" + username) .then(response => response.json()) .then(data => { if (data.is_taken) { alert("Username is already taken. Here are some better suggestions: " + data.suggestions.join(", ")); } else { alert("Username is available."); } }); } let typingTimer; const typingInterval = 800; // e.g. 800ms document.getElementById("usernameInput").addEventListener("keyup", function() { clearTimeout(typingTimer); if (this.value) { typingTimer = setTimeout(() => checkUsernameExistence(this.value), typingInterval); } });
This method uses a combination of pre-defined adjectives, nouns, and random numbers to suggest usernames. The logic is not based on the original username the user typed, but rather tries to suggest something entirely new and “better” based on the predefined lists.
You can further refine the suggestion logic to suit your requirements. For example:
- You could use lists of adjectives and nouns relevant to your application’s theme.
- You could use a database of popular or trending words to generate suggestions.
- You could analyze the input username to detect its theme or sentiment and then suggest related words.
There are countless ways to refine the suggestions, depending on your application’s user base and your creativity!