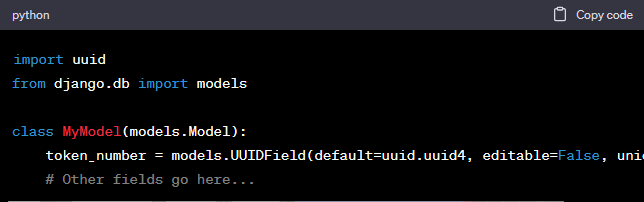
You can create a token number using the `uuid` field in Django. Here’s how you might go about it:
Import UUID Library
First, import the UUID library that comes with Python.
Add UUID Field to Model
You can add a UUID field to your Django model. This field can be used as a token number for any unique identification you might need.
Example Model
import uuid from django.db import models class MyModel(models.Model): token_number = models.UUIDField(default=uuid.uuid4, editable=False, unique=True) # Other fields go here...
Here, `uuid.uuid4` will generate a unique UUID every time a new instance of this model is created. The `editable=False` ensures that the UUID cannot be changed after the object is created, and `unique=True` ensures that each token number is unique across all instances of the model.
Using the Token Number
You can then use this `token_number` as you would any other field in your model. For example, you might use it in your views or serializers if you’re building an API.
Creating an Object with a Token Number
new_object = MyModel.objects.create() print(new_object.token_number) # This will print the unique UUID for this object.
Querying by Token Number
token = uuid.UUID('your-uuid-goes-here') object = MyModel.objects.get(token_number=token)
By using a UUID, you are generating a token that’s almost certainly going to be unique across all objects, and this method avoids the need for you to manually create and manage tokens yourself.
Please note that the above examples are conceptual and may need to be adjusted to fit your specific application and use case.