Generating User Registration Numbers with Python
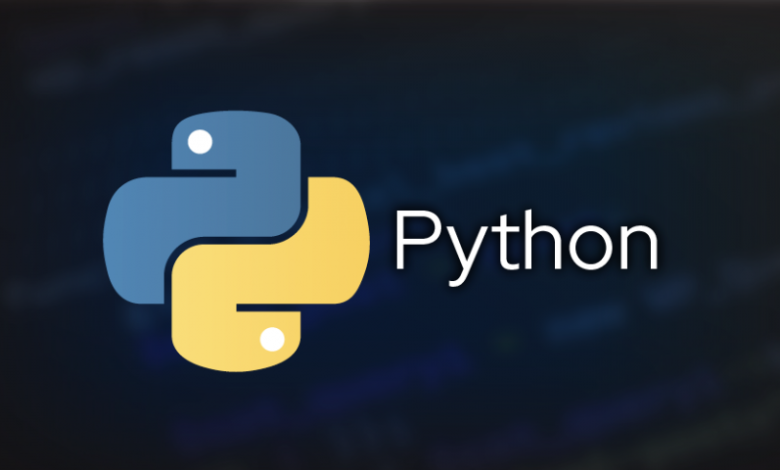
Generating User Registration Numbers with Python
In the modern digital realm, businesses often require a system to uniquely identify their users. A common practice is the generation of user IDs or registration numbers. But what if you want to add a personalized touch to these identifiers?
Today, we’ll explore a Pythonic method of generating unique registration numbers for users, combining personal details and country codes. This method not only ensures uniqueness but also infuses a touch of personalization, making the IDs easier to recall.
The Desired Format
The registration number format we’re aiming for is: AB-{country_code}-{FirstName}{LastName}-ID
.
For instance, for a user named “John Doe” from India (with the country code “0091”) and a database ID of “1”, the registration number will look like this: AB-0091-JD-1
.
Diving into the Code
Let’s delve into the Python function that accomplishes this:
def generate_registration_number(country_code, first_name, last_name, user_id): first_initial = first_name[0].upper() last_initial = last_name[0].upper() registration_number = f"LY-{country_code}-{first_initial}{last_initial}-{user_id}" return registration_number
Code Breakdown:
- Function Definition: Our function,
generate_registration_number
, requires four arguments: country_code, first_name, last_name, and user_id. - Extracting Initials: We harness the power of Python string manipulation to grab the first character from the first and last names. These initials are then converted to uppercase.
- Formatting the Registration Number: Python’s intuitive f-strings come into play, allowing for seamless and readable string formatting.
Utilizing the Function
To employ the function:
full_name = "John Doe" country_code = "0091" first_name, last_name = full_name.split() user_id = 1 reg_num = generate_registration_number(country_code, first_name, last_name, user_id) print(reg_num)
Ensure your data is validated and sanitized. For example, always verify that first_name and last_name contain characters and that user_id is an integer.
Closing Thoughts
Creating unique and memorable identifiers is a breeze with Python. With just a few lines of code, you can create registration numbers that are not only unique but also carry a touch of personalization.
As developers and businesses, this practice can enhance user experience, making identifiers easier to remember. It’s yet another example of how, with the right approach, even mundane tasks can be infused with creativity and efficiency.
Bonus Tip: You can use the feature in django as well. Please check out our django tutorial.
We are a group of volunteers and starting a new scheme in our community.
Your web site offered us with valuable info to work on. You’ve done an impressive job and our whole community will be thankful to you.
Hi! I’m at work browsing your blog from my new iphone 3gs!
Just wanted to say I love reading through your blog and
look forward to all your posts! Keep up the excellent
work!
Thanks so much for setting up the helpful plus pleasurable platform. I am look ahead towards the knowledge as soon as an instance manifests itself! Thanks a lot once again for allowing the platform ready to worldwide community!