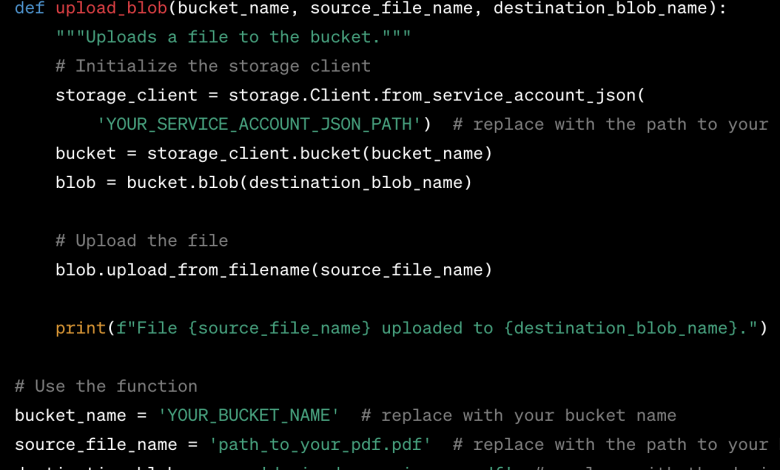
To upload a PDF (or any file) to Google Cloud Storage (GCS) using Python, you can use the Google Cloud Storage Python client library.
Here’s a step-by-step guide to doing so:
Install the Google Cloud Storage client library:
pip install --upgrade google-cloud-storage
Set up Authentication:
Before you can access Google Cloud services, you need to set up authentication.
1. Go to the [Google Cloud Console](https://console.cloud.google.com/).
2. Click the project drop-down and select or create the project that you want to use for accessing GCS.
3. Navigate to **IAM & Admin** > **Service accounts**.
4. Click on **Create Service Account**, provide a name and description, and click **Create**.
5. Grant necessary permissions to the service account. For uploading to GCS, the `Storage Object Creator` role should be sufficient. You can adjust permissions as per your needs.
6. Click **Continue** and then click **Done**.
7. Click on the service account you just created, and under the **Keys** tab, click **Add Key** and choose JSON.
8. This will download a JSON file containing the credentials. Save this file securely, and note its path.
Upload the PDF to GCS:
Here’s a simple Python script to upload a PDF to GCS:
from google.cloud import storage def upload_blob(bucket_name, source_file_name, destination_blob_name): """Uploads a file to the bucket.""" # Initialize the storage client storage_client = storage.Client.from_service_account_json( 'YOUR_SERVICE_ACCOUNT_JSON_PATH') # replace with the path to your JSON credentials bucket = storage_client.bucket(bucket_name) blob = bucket.blob(destination_blob_name) # Upload the file blob.upload_from_filename(source_file_name) print(f"File {source_file_name} uploaded to {destination_blob_name}.") # Use the function bucket_name = 'YOUR_BUCKET_NAME' # replace with your bucket name source_file_name = 'path_to_your_pdf.pdf' # replace with the path to your PDF destination_blob_name = 'desired_name_in_gcs.pdf' # replace with the desired name in GCS upload_blob(bucket_name, source_file_name, destination_blob_name)
Replace `’YOUR_SERVICE_ACCOUNT_JSON_PATH’`, `’YOUR_BUCKET_NAME’`, `’path_to_your_pdf.pdf’`, and `’desired_name_in_gcs.pdf’` with the appropriate values.
After running this script, your PDF will be uploaded to the specified bucket in Google Cloud Storage.