Upload Videos to YouTube: by Django
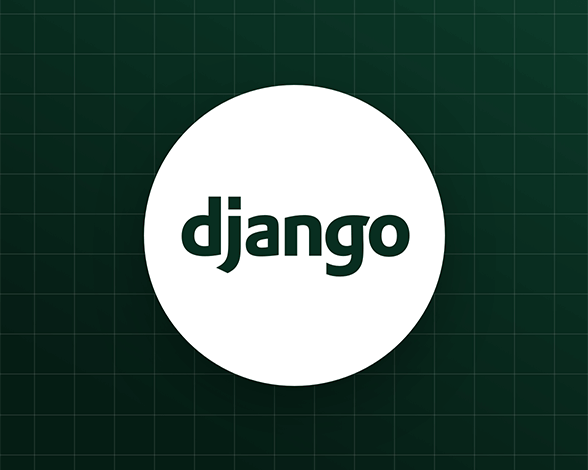
Uploading a video to YouTube from a Django app involves integrating with the YouTube Data API. The process can be divided into a few steps:
Google API Setup
- You need to set up a project on the Google Developers Console.
- Enable the YouTube Data API v3 for the project.
- Create OAuth 2.0 credentials.
Django Setup
- Create a Django model to store video data.
- Create a Django view and form for uploading videos.
- Handle OAuth2 process to authenticate and get permission from the creator.
- Use the API to upload the video.
If you don’t know how to install django, then please read our article. THis will help you create your first django application.
Here’s a simple implementation using Django:
Django Model:
# models.py from django.db import models class Video(models.Model): title = models.CharField(max_length=250) video_file = models.FileField(upload_to='videos/') description = models.TextField()
Form for Video Upload
# forms.py from django import forms from .models import Video class VideoForm(forms.ModelForm): class Meta: model = Video fields = ['title', 'video_file', 'description']
View to Handle Video Upload
First, install the Google client library:
pip install --upgrade google-api-python-client
Then, implement the view
# views.py from django.shortcuts import render, redirect from .forms import VideoForm from google.oauth2.credentials import Credentials from google_auth_oauthlib.flow import InstalledAppFlow from googleapiclient.discovery import build from googleapiclient.http import MediaFileUpload CLIENT_SECRETS_FILE = "path_to_your_client_secret.json" SCOPES = ['https://www.googleapis.com/auth/youtube.upload'] def upload_to_youtube(request): if request.method == 'POST': form = VideoForm(request.POST, request.FILES) if form.is_valid(): video = form.save() # OAuth2 process flow = InstalledAppFlow.from_client_secrets_file(CLIENT_SECRETS_FILE, SCOPES) credentials = flow.run_console() youtube = build('youtube', 'v3', credentials=credentials) body = { 'snippet': { 'title': video.title, 'description': video.description, }, 'status': { 'privacyStatus': 'unlisted' } } media = MediaFileUpload(video.video_file.path, mimetype='video/*', resumable=True) request = youtube.videos().insert(part="snippet,status", body=body, media_body=media) response = request.execute() return redirect('success_page_or_any_other_page') else: form = VideoForm() return render(request, 'upload.html', {'form': form})
HTML Template for Video Upload:
<!-- upload.html --> <form method="post" enctype="multipart/form-data"> {% csrf_token %} {{ form.as_p }} <button type="submit">Upload to YouTube</button> </form>
URL Configuration:
# urls.py from django.urls import path from . import views urlpatterns = [ path('upload/', views.upload_to_youtube, name='upload_to_youtube'), ]
Remember, this is a simplified version. In a real-world scenario, you’d need to handle errors, provide more sophisticated feedback, and probably store the YouTube video ID and other data you might need later. Also, the OAuth2 process in this example is simplified for a command-line approach; in a real application, you’d need a more streamlined web-based flow.