Integrating Redis Cache in django project
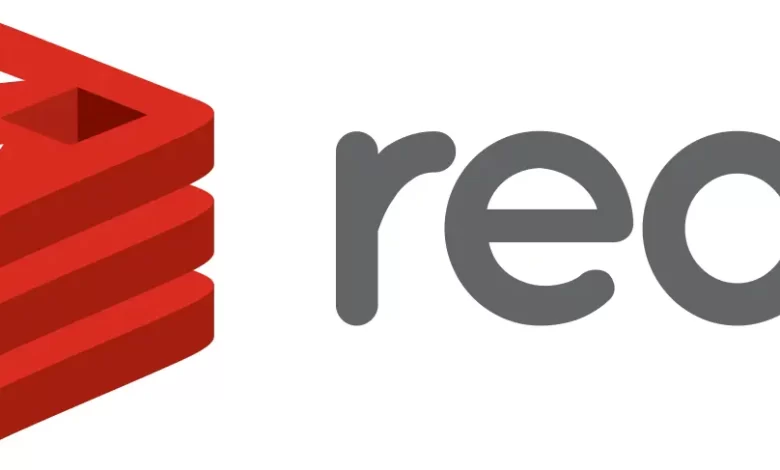
Integrating caching in Django can significantly improve the performance and response time of your web application. Django provides built-in support for various caching backends, such as in-memory caching, file-based caching, database caching, and more. Here’s a step-by-step guide to integrating caching in Django:
Choose a caching backend:
Django supports several caching backends, such as Memcached, Redis, file-based caching, and database caching. Select a backend based on your project requirements and available resources. For example, Redis is a popular choice for its versatility and performance.
Install the required caching backend:
If you choose Memcached or Redis, you’ll need to install the respective server and Python client library. For example, to use Redis, you can install it via pip:
pip install redis
Configure caching in Django settings:
Open your Django project’s settings.py file and configure the caching settings. Set the `CACHES` variable to specify the caching backend and its options. Here’s an example using Redis as the caching backend:
–> settings.py
CACHES = {
'default': {
'BACKEND': 'django_redis.cache.RedisCache',
'LOCATION': 'redis://127.0.0.1:6379/1', # Replace with your Redis server details
'OPTIONS': {
'CLIENT_CLASS': 'django_redis.client.DefaultClient',
}
}
}
Use the cache in your views:
Now that caching is configured, you can use it in your views to cache the output of expensive database queries or other time-consuming operations. Django provides a convenient `cache` decorator to handle caching. Here’s an example:
from django.core.cache import cache
from django.shortcuts import render
def my_view(request):
data = cache.get('my_view_data')
if not data:
data = expensive_database_operation()
cache.set('my_view_data', data, timeout=3600) # Cache for one hour (in seconds)
return render(request, 'my_template.html', {'data': data})
In this example, the view first tries to retrieve the data from the cache. If it’s not found, it performs the expensive operation, caches the result, and returns it. For subsequent requests, the data will be fetched from the cache, reducing the processing time.
Cache template fragments (optional):
Apart from caching views, you can also cache individual template fragments using the `{% cache %}` template tag. This is useful when you have parts of the template that don’t change frequently and can be cached for a certain period.
Consider cache invalidation:
Plan for cache invalidation to ensure that users see the most up-to-date data when it changes. You can manually invalidate cache keys when data is updated or use Django’s cache invalidation mechanisms.
Monitor and optimize:
Monitor your application’s performance with caching enabled to ensure that it’s working effectively. You can use tools like Django Debug Toolbar or cache monitoring tools specific to your chosen caching backend.
Remember that caching is a powerful tool, but it should be used judiciously. Not all data is suitable for caching, and caching strategies may vary depending on your application’s needs. Always test and profile your application to ensure that caching is improving performance as expected.