How to use functions in javascript ?
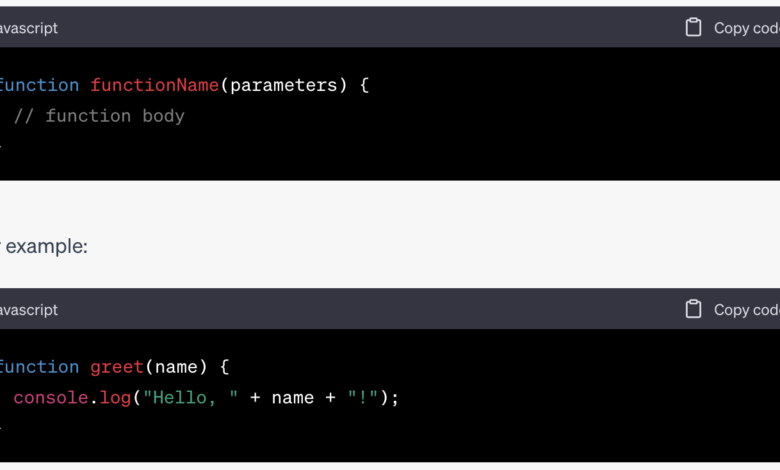
Functions in JavaScript allow you to encapsulate a block of code that can be executed multiple times with different inputs and produce a desired output. Here’s how you can define and use functions in JavaScript:
Function Definition:
To define a function, you use the `function` keyword followed by the function name, a pair of parentheses `()`, and a pair of curly braces `{}` to enclose the function body. The function body contains the code that will be executed when the function is called. Here’s the syntax:
“`javascript
function functionName(parameters) {
// function body
}
“`
For example:
“`javascript
function greet(name) {
console.log("Hello, " + name + "!");
}
“`
Function Call:
To call or invoke a function, you simply write the function name followed by a pair of parentheses `()`. If the function has parameters, you pass the corresponding values inside the parentheses. Here’s the syntax:
“`javascript
functionName(arguments);
“`
For example:
“`javascript
greet("John"); // Output: Hello, John!
“`
Return Statement:
Functions can also return a value using the `return` statement. This allows the function to calculate a result and provide it back to the code that called the function. The `return` statement ends the function execution and sends the specified value back. Here’s an example:
“`javascript
function add(a, b) {
return a + b;
}
“`
I hope this helps you understand how to use functions in JavaScript!