Learn global & block level scope
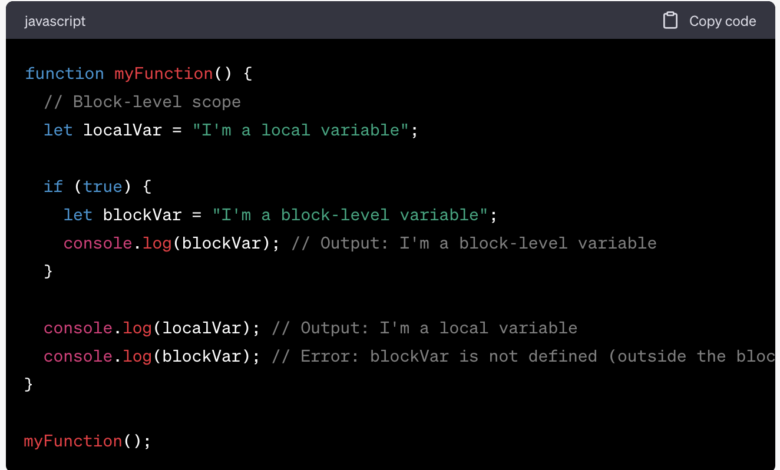
Understanding global and block-level scope in JavaScript is crucial for writing well-organized and efficient code. Here’s an explanation of global and block-level scope and some suggestions on how to learn more about them:
Global Scope:
Variables declared outside of any function or block have global scope. They can be accessed from anywhere in your JavaScript code, including other functions or blocks. However, it is generally recommended to minimize the use of global variables to avoid potential naming conflicts and maintain code clarity.
Example:
“`javascript
// Global scope
let globalVar = "I'm a global variable";
function myFunction() {
console.log(globalVar); // Accessible inside functions
}
myFunction(); // Output: I’m a global variable
console.log(globalVar); // Output: I’m a global variable
“`
Block-Level Scope:
Block-level scope refers to variables declared within a block, such as inside a function, loop, or conditional statement. These variables are only accessible within the block where they are defined. Block-level scope was introduced in ES6 (ECMAScript 2015) using the `let` and `const` keywords. Variables declared with `let` or `const` have block-level scope.
Example:
“`javascript
function myFunction() {
// Block-level scope
let localVar = "I'm a local variable";
if (true) {
let blockVar = “I’m a block-level variable”;
console.log(blockVar); // Output: I’m a block-level variable
}
console.log(localVar); // Output: I’m a local variable
console.log(blockVar); // Error: blockVar is not defined (outside the block)
}
myFunction();
“`
Learning Resources:
To enhance your understanding of global and block-level scope in JavaScript, you can explore the following resources:
– MDN Web Docs: The MDN documentation provides comprehensive information about JavaScript’s global and block-level scope. You can refer to the following pages:
– Global Scope: [MDN Global Scope](https://developer.mozilla.org/en-US/docs/Glossary/Global_scope)
– Block Scope: [MDN Block Scope](https://developer.mozilla.org/en-US/docs/Glossary/Block_scope)
– JavaScript tutorials and courses: Online tutorials and courses, such as those available on FreeCodeCamp, Codecademy, or Udemy, offer structured learning materials that cover the concepts of scope in JavaScript. Look for topics like variable scope, function scope, and block scope.
– JavaScript books: Books like “Eloquent JavaScript” by Marijn Haverbeke or “You Don’t Know JS” series by Kyle Simpson delve into the intricacies of JavaScript and provide in-depth explanations of scope.
By studying these resources and practicing with code examples, you’ll develop a solid understanding of global and block-level scope in JavaScript, enabling you to write more organized and efficient code.