Master if-else conditions using JavaScript
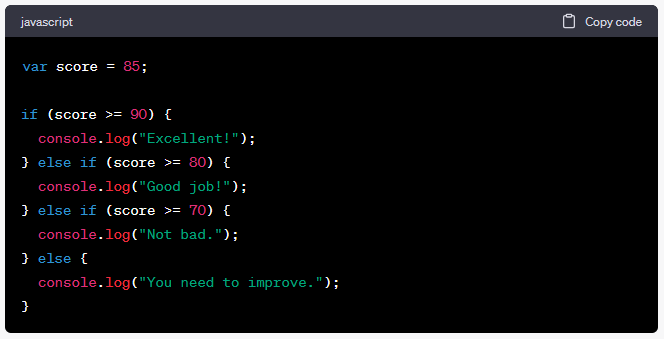
In JavaScript, you can use if-else statements to create conditional logic. The basic syntax for an if-else statement is as follows:
Basic Syntax:
if (condition) {
// code to be executed if the condition is true
} else {
// code to be executed if the condition is false
}
Example:
Here’s an example to illustrate how if-else conditions work:
var age = 18;
if (age >= 18) {
console.log("You are eligible to vote.");
} else {
console.log("You are not eligible to vote yet.");
}
Practical Example:
In this example, the code checks if the `age` variable is greater than or equal to 18. If the condition evaluates to true, it executes the code inside the `if` block, which displays “You are eligible to vote.” Otherwise, if the condition is false, it executes the code inside the `else` block, which displays “You are not eligible to vote yet.”
You can also include multiple `else if` statements to check for additional conditions. Here’s an expanded example:
var score = 85;
if (score >= 90) {
console.log("Excellent!");
} else if (score >= 80) {
console.log("Good job!");
} else if (score >= 70) {
console.log("Not bad.");
} else {
console.log("You need to improve.");
}
In this case, the code evaluates the `score` variable against different conditions and prints different messages based on the score range.
Remember, the `else if` and `else` blocks are optional. If you only need to check a single condition, you can omit the `else if` and `else` parts. Additionally, you can nest if-else statements within each other to create more complex logic.